API Development: Build Efficient APIs Quickly
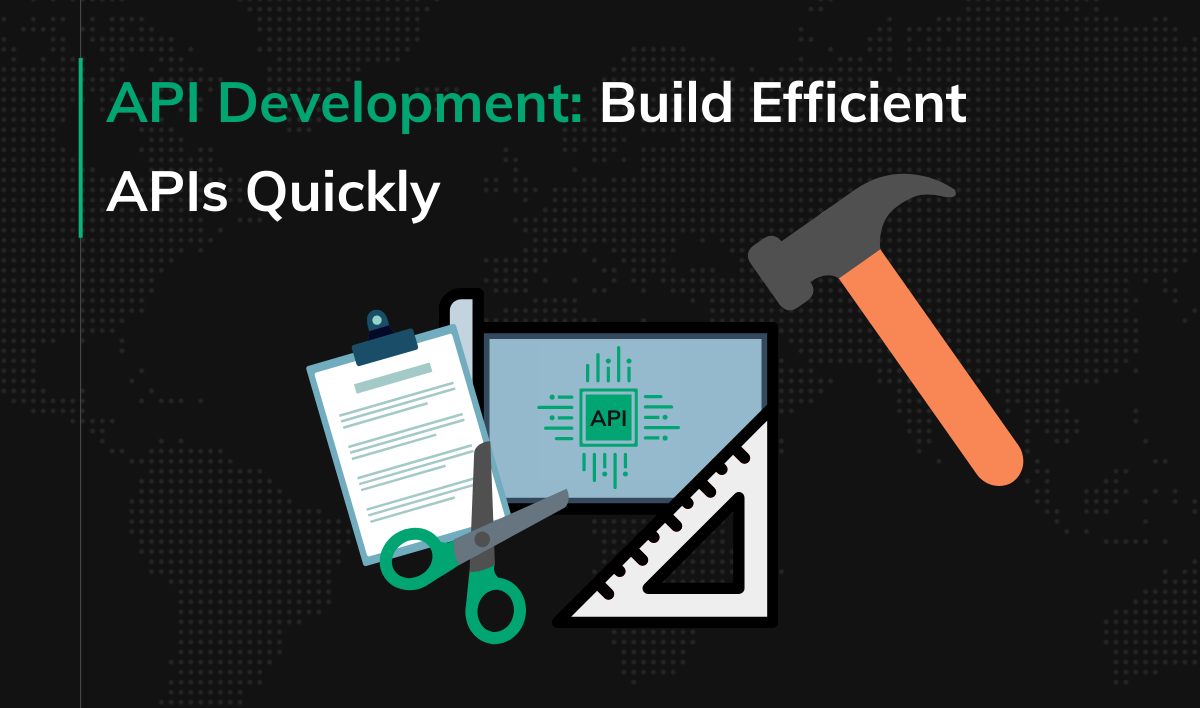
APIs are the backbone of today’s digital world. They let different software systems talk to each other. If you’ve ever used an app to check your bank balance or booked a ride on your phone, you’ve used an API. In this guide, we’ll explore how to build efficient APIs quickly. We’ll focus on best practices and tools, to keep things actionable. Let’s dive in!
Key Points
|
Introduction to API Development
APIs act as bridges between different software applications. They allow systems to interact and share data easily. APIs set the rules and protocols for communication, ensuring systems work together smoothly.
What Is an API?
An API, or Application Programming Interface, is like a waiter in a restaurant. Imagine you order a meal; the waiter takes your request to the kitchen, and then brings your food back to you. Similarly, an API takes a user’s request to a system and returns the response back to the user. This process enables different software applications to interact without needing to understand each other’s internal workings.
The Role of APIs in Technology
APIs enhance functionality by letting developers integrate existing features into new apps. Instead of building a mapping system from scratch, for example, a developer can use the Google Maps API to embed maps. This connectivity is crucial in modern software development. It allows services to communicate, share data, and offer richer user experiences.
Key API Terminologies
Understanding APIs involves some essential terms:
- API Endpoints: URLs where APIs can access resources.
- API Keys: Unique codes that identify and authenticate the calling program.
- API Gateway: A server that acts as a single entry point for a set of APIs.
- OAuth: An open standard for access delegation, commonly used for token-based authentication.
- REST and SOAP APIs: Protocols that define how APIs are structured and how they communicate.
Understanding APIs
APIs come with architectures that define their structure and behavior. Good documentation and robust security practices are also crucial components of effective API development.
API Architecture
API architecture refers to the blueprint of how an API functions, including its structure and organization. There are different architectural styles:
- REST(Representational State Transfer): Uses standard HTTP methods and is stateless.
- SOAP(Simple Object Access Protocol): Relies on XML messaging and has built-in error handling.
- Microservices Architecture: A modern approach in which applications are built as a collection of small, independent services. Each service runs on its own and communicates through APIs. This design makes applications more scalable and easier to manage. Microservices allow developers to update parts without affecting the whole system.
By understanding these architectural styles—REST, SOAP, and Microservices—you can choose the one that best fits your project’s needs. Each has its own advantages, so consider your requirements carefully.
API Documentation
Clear and comprehensive documentation is vital for helping developers understand how to use your API. Good documentation should include:
- Endpoint Descriptions: Detailed explanations of each endpoint.
- Request and Response Examples: Sample code snippets.
- Error Codes and Messages: Information on how to handle errors.
Tools like Swagger, which utilizes the OpenAPI Specification, assist in creating interactive and up-to-date API documentation, making it easier for developers to explore and test your API.
API Security Practices
Security is paramount in API development to protect data and ensure only authorized users have access.
- Authentication and Authorization: Verify the identity of users and control what they can access.
- Role and Route-Based Permissions: Assign permissions based on user roles and specific routes.
- SSL/TLS Encryption: Encrypt data in transit to prevent interception and tampering.
Implementing strong security measures builds trust with users. It keeps sensitive information safe. This is especially important when integrating APIs in banking. Other high-security sectors need this level of protection too.
Types of APIs
There are various types of APIs, each suited for different use cases and offering unique advantages.
REST APIs
REST APIs follow the REST architectural style, using standard HTTP methods like GET, POST, PUT, and DELETE.
Principles of RESTful Architecture:
- Statelessness: Each request is independent and doesn’t rely on previous interactions.
- Client-Server Separation: The client interface is separate from the server functionality.
- Uniform Interface: Consistent method of communication, making it easier to understand and use.
Use Cases and Benefits:
- Web Services: Ideal for web applications due to their simplicity and scalability.
- Easy Implementation: Straightforward to develop and consume.
- Flexibility: Can handle a variety of data formats like JSON and XML.
SOAP APIs
SOAP is a protocol that relies on XML for messaging and offers built-in error handling.
SOAP Protocol and Applications:
- Structured Messaging: Uses XML schemas for defining message formats.
- Enterprise-Level Transactions: Suited for complex transactions that require high security.
Comparing SOAP with REST:
- Complexity: SOAP is more rigid and complex than REST.
- Security: Offers advanced security features like WS-Security.
- Performance: Generally slower due to XML parsing and heavier payloads.
GraphQL APIs
GraphQL is a query language developed by Facebook that allows clients to request exactly the data they need.
Advantages Over Traditional APIs:
- Efficient Data Retrieval: Reduces over-fetching and under-fetching of data.
- Single Endpoint: All data is accessed through a single endpoint, simplifying the API structure.
WebSocket APIs
WebSocket APIs enable real-time, two-way communication between client and server.
Suitable Use Cases:
- Live Chat Applications: Facilitates instant messaging.
- Real-Time Updates: Ideal for live feeds, gaming, and collaborative tools.
Other API Types
- Open APIs (Public APIs): Available for public use without restrictions.
- Private APIs: Used internally within an organization.
- Partner APIs: Shared with specific business partners.
- Composite APIs: Combine multiple API calls into a single request to improve performance.
API Design and Architecture
Designing an effective API requires thoughtful consideration of various factors to meet both current and future needs.
Choosing an Architectural Style
When selecting an architectural style, consider:
- Functional Requirements: What the API needs to accomplish.
- Non-Functional Requirements: Performance, scalability, and security needs.
- Client Needs: How clients will use the API and what data they require.
Comparing Styles:
- REST: Ideal for general-purpose APIs with standard CRUD operations.
- SOAP: Suitable for secure, enterprise-level transactions.
- GraphQL: Best when clients need precise data queries.
- WebSockets: Necessary for real-time data communication.
Data Formats and Protocols
Choosing the right data format and protocol ensures efficient communication.
- JSON (JavaScript Object Notation): Lightweight and easy to parse; widely used with REST APIs.
- XML (eXtensible Markup Language): More verbose; used primarily with SOAP APIs.
Similarly, selecting the appropriate protocols is crucial. Protocols and standards like HTTP, HTTPS, and WebSocket define how data is transmitted and secured, working alongside data formats to facilitate smooth and secure communication between clients and servers.
API Gateway and Microservices
An API Gateway acts as a single entry point for your APIs. It handles tasks like authentication, routing, and rate limiting. This simplifies how clients interact with your application because they don’t need to manage multiple endpoints.
Now, let’s talk about Microservices. This is an architectural approach where you build your application as a collection of small, independent services. Each service runs on its own and communicates with others through APIs.
By combining an API Gateway with Microservices, you gain several benefits:
- Scalability: You can scale each service independently based on demand.
- Maintainability: It’s easier to update or fix one service without affecting the whole system.
- Flexibility: Teams can choose the best technologies for their specific services.
Usability and Security
Balancing usability and security is crucial when designing your API. You want developers to find your API easy to use, which encourages adoption. At the same time, you need robust security to protect data and comply with regulations.
Let’s look at how to achieve this balance:
- Ease of Use: Simplify your API interface to make it intuitive. Use clear and consistent naming conventions. Provide detailed documentation with examples. An easy-to-use API attracts more developers and reduces integration time.
- Robust Security Measures: Implement strong authentication methods like OAuth 2.0 and API keys. Encrypt data using SSL/TLS protocols to ensure secure communication. Regularly update your security protocols to guard against new threats.
By designing with both in mind, you create APIs that are secure yet developer-friendly.
The API Development Process
Developing an API involves several key stages, from initial planning to deployment and feedback.
Planning Your API
Define the scope and requirements:
- Functional Requirements: List all the features and operations the API should support.
- Non-Functional Requirements: Consider performance targets, security protocols, and scalability needs.
Early planning sets a clear roadmap for development and helps avoid scope creep.
Designing the API
Create detailed specifications:
- API Endpoints: Define the URLs and HTTP methods for each operation.
- Request and Response Formats: Decide on data formats (e.g., JSON, XML) and structure.
- Error Handling: Establish standard error codes and messages.
A well-thought-out design ensures consistency and makes the API easier to use and maintain.
Development Stage
Choose appropriate tools and technologies:
- Technology Stack: Select programming languages, frameworks, libraries, and AI agents that suit your needs.
- Version Control: Use platforms like GitHub to track changes and collaborate with team members.
- Coding Best Practices: Write clean, modular code with proper documentation.
Implementing these simple practices leads to more reliable and maintainable APIs.
Testing and Debugging
Ensure your API functions correctly and securely:
- Unit Testing: Test individual components for expected functionality.
- Integration Testing: Verify that different parts of the API work together seamlessly.
- Security Testing: Use tools to scan for vulnerabilities and ensure compliance with security standards.
- Testing Tools: Utilize Postman, SoapUI, or similar tools for efficient testing.
Thorough testing identifies issues early, reducing the risk of problems after deployment.
Deployment
Prepare your API for public use:
- Deployment Strategies: Choose between cloud services, on-premises servers, or hybrid solutions.
- Continuous Integration/Continuous Deployment (CI/CD): Automate the deployment process for faster releases.
- Scalable Infrastructure: Ensure your hosting environment can handle increased load as your API grows.
Effective deployment strategies contribute to better performance and user satisfaction.
Gathering User Feedback
User feedback is invaluable for ongoing improvement:
- Feedback Channels: Provide ways for users to report issues or suggest features.
- Analytics: Monitor usage patterns to understand how the API is being used.
- Iterative Development: Continuously update and refine the API based on feedback.
Quick Tips:
- Set Up Regular Check-Ins: Schedule periodic meetings or calls with key users to gather direct feedback.
- Use In-App Surveys: Implement short surveys within your developer portal to collect user opinions without much effort on their part.
- Create a Feedback Loop: Let users know how their feedback has influenced changes. This encourages more engagement and builds trust.
Engaging with users helps build a better product that meets their needs. By actively seeking feedback and acting on it, you make your API more effective and user-friendly.
Best Practices for API Development
Implementing best practices enhances the quality and usability of your API.
API Design Principles
- Consistency and Stability: Maintain consistent naming conventions and behaviors across endpoints.
- Clear Naming Conventions: Use intuitive and descriptive names for resources and actions.
- Extensibility and Future-Proofing: Design the API to accommodate future features without breaking existing functionality.
These principles make your API easier to use and reduce the likelihood of errors.
Enhancing Developer Experience
Make your API appealing to other developers:
- Comprehensive Documentation: Provide clear instructions, examples, and tutorials.
- SDKs and Libraries: Offer software development kits in popular programming languages to simplify integration.
- Modification Timestamps: Include timestamps to help clients manage caching and updates.
A positive developer experience encourages adoption and integration of your API.
Performance Optimization
Ensure your API performs well under various conditions:
- Monitoring Performance Metrics: Track response times, throughput, and error rates.
- Reliability Under Heavy Load: Conduct load testing to ensure the API remains stable during high traffic.
- Throttling Mechanisms: Implement rate limiting to prevent abuse and maintain performance.
Optimizing performance leads to a better user experience and more reliable service.
Testing and Mocking
Robust testing is essential for quality assurance:
- Thorough Testing: Cover all possible use cases and scenarios.
- Mocking: Use mock servers to simulate API responses, allowing testing without affecting the production environment.
These practices help identify and fix issues before they impact users.
Use of Throttling
Manage API usage effectively:
- Prevent Abuse: Set limits on the number of requests to protect against denial-of-service attacks.
- Fair Usage Policies: Ensure resources are available to all users by preventing any single user from consuming excessive resources.
Throttling helps maintain service quality and availability for all users.
API Tools and Platforms
Selecting the right tools and platforms accelerates development and enhances the quality of your API.
Development and Testing Tools
Postman
Postman simplifies API development and testing with its user-friendly interface.
- Construct and Send Requests: Easily create and modify HTTP requests.
- Automated Testing: Create test suites to validate API responses.
- Collaboration: Share collections and environments with team members.
- Environment Variables: Manage different settings for development, staging, and production.
Using Postman streamlines testing and ensures your API endpoints function correctly before deployment.
Swagger and OpenAPI
Swagger and the OpenAPI Specification help in designing and documenting APIs.
- Interactive Documentation: Generate documentation that allows developers to try out API calls directly.
- Design First Approach: Plan your API structure before coding, reducing inconsistencies.
- Code Generation: Automatically create server stubs and client SDKs in various languages.
These tools enhance clarity and make your API more accessible to other developers.
GitHub
GitHub facilitates version control and collaboration.
- Track Changes: Maintain a history of code modifications.
- Collaborate: Work with others through pull requests and code reviews.
- Integrate Tools: Connect with CI/CD pipelines and other development tools.
GitHub ensures code quality and fosters teamwork in API development projects.
API Management Platforms
Apigee
Apigee (now part of Google) provides comprehensive tools for API management.
- Analytics: Monitor API usage and performance.
- Security: Implement policies for authentication and authorization.
- Monetization: Create billing plans to monetize your API.
- Developer Portal: Offer a platform for developers to access documentation and support.
Apigee helps manage the entire API lifecycle, making it suitable for both small and enterprise-level projects.
RapidAPI
RapidAPI is a marketplace connecting API providers with developers.
- Publish APIs: Increase visibility and reach a broader audience.
- Manage Subscriptions: Handle access levels and billing efficiently.
- Provide Testing Tools: Allow developers to test APIs directly on the platform.
Using RapidAPI can accelerate adoption and simplify distribution.
API-Platform
API-Platform is an open-source framework for building API-driven projects.
- Framework Support: Compatible with Symfony and other PHP frameworks.
- REST and GraphQL Support: Build APIs using your preferred style.
- Out-of-the-Box Features: Includes pagination, validation, and filtering.
- Customization: Highly extensible to meet specific needs.
API-Platform speeds up development by handling common functionalities.
Serverless Architecture
Serverless architecture enables you to run applications without managing servers.
Benefits:
- Cost Efficiency: Pay only for actual usage.
- Automatic Scaling: Adjust resources based on demand.
- Reduced Maintenance: Focus on code rather than infrastructure.
Platforms:
- AWS Lambda: Integrates with AWS services; supports multiple languages.
- Azure Functions: Offers seamless integration with Azure services and supports continuous deployment.
Serverless platforms are ideal for building scalable APIs quickly, especially when dealing with variable loads.
Additional Tools
APIMatic
APIMatic helps generate SDKs for your APIs.
- Multi-Language Support: Create client libraries in various programming languages.
- Ease of Integration: Simplify the process for developers to use your API.
- Automatic Updates: Keep SDKs consistent with API changes.
Providing SDKs reduces integration time and encourages adoption.
SoapUI
SoapUI is designed for testing SOAP and REST APIs.
- Functional Testing: Automate testing to validate API behavior.
- Load Testing: Assess performance under heavy traffic.
- Security Testing: Identify vulnerabilities and ensure compliance.
SoapUI ensures your API is robust and secure before it goes live.
Sandbox Environments
Sandbox environments allow safe testing.
- Isolated Testing: Experiment without affecting production data.
- User Acceptance Testing: Gather feedback before full deployment.
- Integration Testing: Verify compatibility with other systems.
Using a sandbox minimizes risks and improves the quality of your API.
API Cost Considerations
Understanding the costs involved in API development helps in budgeting and resource allocation.
Factors Influencing Development Cost
- Complexity and Functionality: More features require more development time and resources.
- Technology Stack Selection: Some technologies have licensing fees; others are open-source.
- Development Team Location and Expertise: Labor costs vary by region and skill level.
Recognizing these factors helps in creating a realistic budget.
Budgeting and Resource Allocation
Plan for expenses throughout the API lifecycle:
- Development Costs: Initial coding and testing.
- Infrastructure Costs: Hosting, servers, and network resources.
- Maintenance Costs: Ongoing updates and support.
Allocating resources appropriately ensures smooth development and operation.
Optimizing Costs
- Vendor Management Solutions: Use existing platforms to reduce development time.
- Third-Party Developers: Outsource to experts when necessary.
- Interconnected Ecosystems: Leverage existing services to add functionality without additional development.
These strategies can help save money without compromising quality.
Revenue Generation
APIs can be a significant source of income for your business. By offering access to your API, you open up new revenue streams.
- Monetizing APIs: Charge for API access using subscription models or pay-per-use pricing. For example, you can offer different plans based on usage levels.
- Setting Up for Income: Implement API keys or tokens to control access. Use analytics to track usage and set fair pricing.
- Quick Time to Market: The faster your API is available, the sooner it can generate revenue. Focus on core features to launch quickly.
While income is a strong motivator, APIs also enhance your product ecosystem. They allow others to build on your services, expanding your reach. So, planning for monetization makes your API a valuable asset for your business.
Real-Time Analysis
Use analytics to make informed decisions.
- Budget Allocation: Adjust spending based on usage data and performance metrics.
- Resource Scaling: Increase or decrease resources in response to demand.
Real-time data helps optimize both performance and costs.
API Monitoring and Maintenance
Ongoing monitoring and maintenance are essential for long-term success.
Importance of Post-Deployment Monitoring
- Performance Tracking: Ensure the API continues to meet performance benchmarks.
- Reliability: Maintain high availability to meet user expectations.
Continuous monitoring helps detect and address issues promptly.
Performance Metrics to Track
- Response Times: Measure how quickly your API responds to requests.
- Server Resource Usage: Monitor CPU and memory to prevent overloads.
- Error Rates: Identify and fix issues causing failures.
Tracking these metrics ensures your API remains efficient and reliable.
Tools for Monitoring
Keeping an eye on your API is crucial. Different types of tools help you monitor performance and ensure reliability.
- Performance Monitoring Tools: These track response times and error rates. They alert you to issues so you can fix them fast.
- Logging Systems: Logging records events and errors. It’s essential for troubleshooting and understanding user behavior.
- Automated Testing Tools: Regular tests check that your API endpoints work correctly. They help catch problems before users do.
- Analytics Platforms: Analytics show how users interact with your API. Insights from analytics guide improvements.
By using these types of tools, you maintain your API’s health. They help you provide a better experience for your users.
Identifying and Resolving Bottlenecks
- Real-Time Data Analysis: Use dashboards to visualize performance metrics.
- Infrastructure Adjustments: Scale resources as needed to handle load.
Proactive management prevents performance degradation.
Maintenance Best Practices
- Regular Updates: Apply patches and updates to keep the API secure.
- User Feedback: Continuously improve based on user experiences and suggestions.
Ongoing maintenance ensures your API remains relevant and effective.
Building APIs is often just the first step—ensuring they integrate seamlessly with other systems can present even greater challenges. To dive deeper into the complexities of software integration and how to overcome them, read our guide: Beyond ‘Just integrate it’: Real challenges of software integration.
And one more thing: we’ve been diving deep into API development and how crucial it is for modern software. But here’s an interesting twist: AI is shaking things up, especially for SaaS companies. As AI agents handle more tasks, SaaS applications risk becoming commoditized, serving merely as interchangeable tools that AI can swap in and out as needed. Curious about this shift? Check out our article on how AI agents might turn SaaS into just an… API.
Conclusion
Building efficient APIs is essential in today’s digital landscape. By following best practices, you can create APIs that are secure, scalable, and user-friendly. Whether you’re in finance, healthcare, or any other industry, APIs enable innovation and connectivity. Start applying these principles today to build APIs that make a difference.